Multiplication is a fundamental arithmetic operation in assembly language programming. This blog post explores an 8086 assembly language program that performs the multiplication of two 8-bit numbers using the MUL
instruction. The result is stored in a 16-bit destination.
data segment a db 09h b db 02h c dw ? data ends code segment assume cs:code, ds:data start: mov ax,data mov ds,ax mov ax,0000h mov bx,0000h mov al,a mov bl,b mul b mov c,ax int 3 code ends end start
Step-by-Step Explanation:
1. Data Segment Initialization
a db 09h
stores the first operand (9 in decimal) as an 8-bit value.b db 02h
stores the second operand (2 in decimal) as an 8-bit value.c dw ?
reserves a word (16-bit) for storing the result.
2. Code Execution Flow
mov ax, data
andmov ds, ax
: Initialize the data segment by loading its address into the AX register and moving it to DS.mov ax, 0000h
andmov bx, 0000h
: Clear AX and BX registers.mov al, a
: Load the first operand into AL.mov bl, b
: Load the second operand into BL.mul b
: Perform the multiplication of AL by BL, storing the result in AX (AH:AL).mov c, ax
: Store the result in variablec
.int 3
: Generates a breakpoint interrupt to terminate the program execution.
Flowchart:
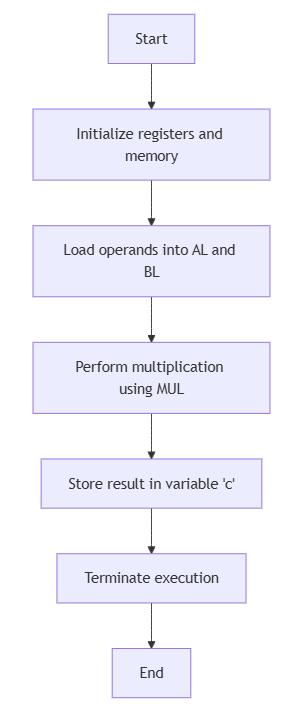
Overall Process:
- The program initializes registers and sets up pointers to the data segment.
- It loads the operands into appropriate registers.
- The
MUL
instruction is executed to perform the multiplication, storing the result inAX
. - The result is stored in the destination variable.
- The program terminates after executing the multiplication operation.
Output
C:\TASM>masm an8mul.asm Microsoft (R) Macro Assembler Version 5.00 Copyright (C) Microsoft Corp 1981-1985, 1987. All rights reserved. Object filename [an8mul.OBJ]: Source listing [NUL.LST]: Cross-reference [NUL.CRF]: 50402 + 450254 Bytes symbol space free 0 Warning Errors 0 Severe Errors C:\TASM>link an8mul.obj Microsoft (R) Overlay Linker Version 3.60 Copyright (C) Microsoft Corp 1983-1987. All rights reserved. Run File [AN8MUL.EXE]: List File [NUL.MAP]: Libraries [.LIB]: LINK : warning L4021: no stack segment C:\TASM>debug an8mul.exe -g AX=0012 BX=0002 CX=002A DX=0000 SP=0000 BP=0000 SI=0000 DI=0000 DS=0B97 ES=0B87 SS=0B97 CS=0B98 IP=0019 NV UP EI PL NZ NA PO NC 0B98:0019 CC INT 3 -d 0B97:0000 0B97:0000 09 02 12 00 00 00 00 00-00 00 00 00 00 00 00 00 ................ 0B97:0010 B8 97 0B 8E D8 B8 00 00-BB 00 00 A0 00 00 8A 1E ................ 0B97:0020 01 00 F6 26 01 00 A3 02-00 CC FF 2A E4 50 B8 FD ...&.......*.P.. 0B97:0030 05 50 FF 36 24 21 E8 77-63 83 C4 06 FF 36 24 21 .P.6$!.wc....6$! 0B97:0040 B8 0A 00 50 E8 47 5E 83-C4 04 5E 8B E5 5D C3 90 ...P.G^...^..].. 0B97:0050 55 8B EC 81 EC 84 00 C4-5E 04 26 80 7F 0A 00 74 U.......^.&....t 0B97:0060 3E 8B 46 08 8B 56 0A 89-46 FC 89 56 FE C4 5E FC >.F..V..F..V..^. 0B97:0070 26 8A 47 0C 2A E4 40 50-8B C3 05 0C 00 52 50 E8 &.G.*[email protected]. -q
Understanding the Memory Dump
Memory Address | Value (Hex) | Binary Representation | Decimal Equivalent | Explanation |
---|---|---|---|---|
0B97:0000 | 09 02 | 0000 1001 0000 0010 | 9, 2 | Input values a = 9 , b = 2 |
0B97:0002 | 12 | 0001 0010 | 18 | Result of 9 * 2 = 18 |
Summary of Output
- The original numbers
09h
(9 in decimal) and02h
(2 in decimal) are processed. - The multiplication operation computes
9 * 2 = 18
, which is stored inc
. - The final result stored in memory is
12h
(18 in decimal).
None of your programs are running in tasm for win 10. File is compiling but output is blank
Yes, Change ur career u are a failure. Children are suffering by learning 43 year old language and you are adding to that suffering.
He helped as to pass off our clg lab sessions. I am a pro in C, but I cant do this becoz i started my programming carrier for embedded right from C.