In this blog post, we will explore an 8086 assembly language program designed to sort a list of numbers in ascending order. Sorting is a fundamental operation in computer science, and understanding how to implement it at the assembly level provides valuable insights into low-level programming and processor operations.
The following assembly program sorts an array of numbers in ascending order using the bubble sort algorithm:
DATA SEGMENT STRING1 DB 99H,12H,56H,45H,36H DATA ENDS CODE SEGMENT ASSUME CS:CODE,DS:DATA START: MOV AX,DATA MOV DS,AX MOV CH,04H UP2: MOV CL,04H LEA SI,STRING1 UP1: MOV AL,[SI] MOV BL,[SI+1] CMP AL,BL JC DOWN MOV DL,[SI+1] XCHG [SI],DL MOV [SI+1],DL DOWN: INC SI DEC CL JNZ UP1 DEC CH JNZ UP2 INT 3 CODE ENDS END START
Step-by-Step Explanation:
1. Data Segment:
STRING1 DB 99H,12H,56H,45H,36H
: Defines an array of bytes containing the numbers to be sorted: 99H, 12H, 56H, 45H, and 36H.
2. Code Segment:
MOV AX,DATA
andMOV DS,AX
: Initialize the data segment by loading the address of the data segment into the AX register and then moving it to the DS register.MOV CH,04H
: Sets the outer loop counter (CH) to 4, representing the number of passes needed for the bubble sort algorithm.
3. Outer Loop (UP2
):
MOV CL,04H
: Initializes the inner loop counter (CL) to 4 for each pass.LEA SI,STRING1
: Loads the effective address of the first element of the array into the SI register.
4. Inner Loop (UP1
):
MOV AL,[SI]
andMOV BL,[SI+1]
: Load the current element and the next element of the array into the AL and BL registers, respectively.CMP AL,BL
: Compares the current element (AL) with the next element (BL).JC DOWN
: If the current element is less than the next element, no swap is needed, and the program jumps to theDOWN
label.MOV DL,[SI+1]
,XCHG [SI],DL
, andMOV [SI+1],DL
: If the current element is greater than the next element, these instructions swap the two elements to arrange them in ascending order.
5. Loop Control:
INC SI
: Increments the SI register to point to the next pair of elements in the array.DEC CL
andJNZ UP1
: Decrements the inner loop counter and jumps back to theUP1
label if CL is not zero, continuing the inner loop.DEC CH
andJNZ UP2
: Decrements the outer loop counter and jumps back to theUP2
label if CH is not zero, initiating another pass through the array.
6. Program Termination:
INT 3
: Generates a breakpoint interrupt to terminate the program execution.
Flowchart:
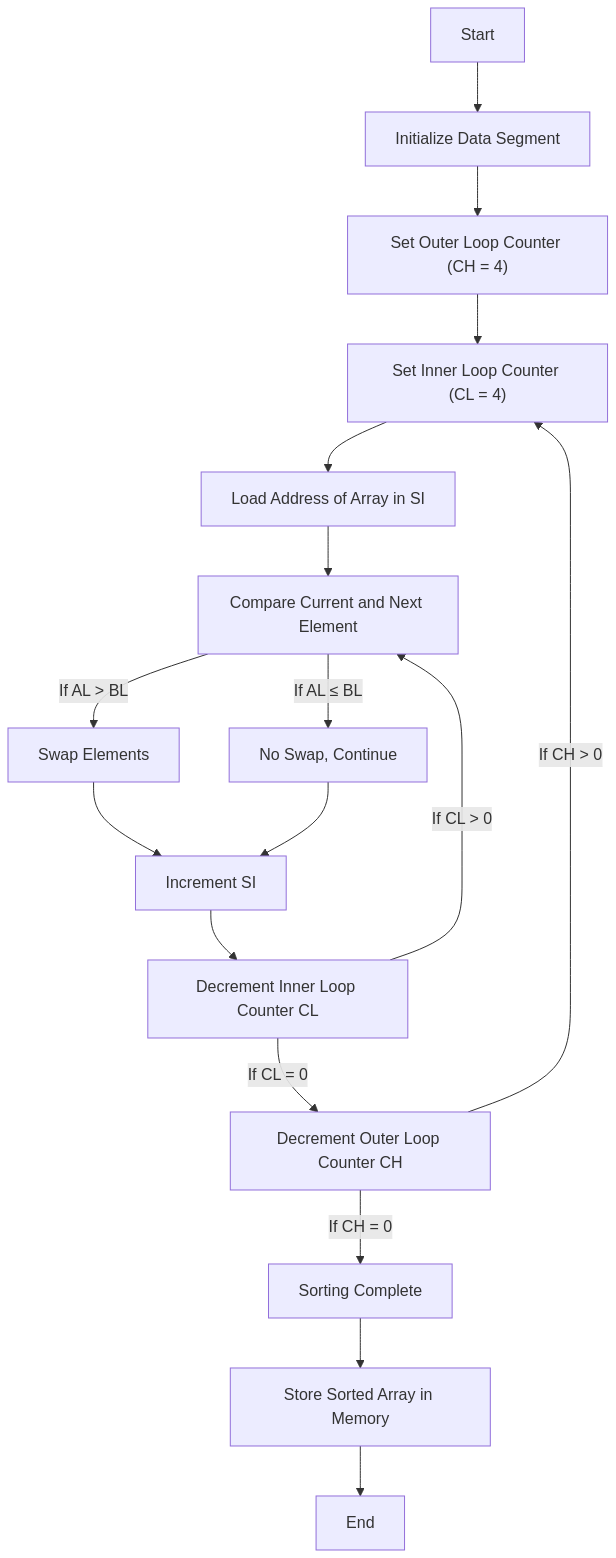
Overall Process:
- The program initializes loop counters and sets up registers for sorting.
- It iterates through the array multiple times to ensure all numbers are sorted in ascending order.
- For each pair of adjacent numbers, it compares them to check if they are in the correct order.
- If the current number is larger than the next number, it swaps them to move the smaller number forward.
- After completing all passes, the numbers are fully sorted in ascending order.
- The sorted numbers remain stored in memory, and the program terminates.
Output
C:\TASM>masm AMPE10.asm Microsoft (R) Macro Assembler Version 5.00 Copyright (C) Microsoft Corp 1981-1985, 1987. All rights reserved. Object filename [AMPE10.OBJ]: Source listing [NUL.LST]: Cross-reference [NUL.CRF]: 49452 + 414900 Bytes symbol space free 0 Warning Errors 0 Severe Errors C:\TASM>link AMPE10.obj Microsoft (R) Overlay Linker Version 3.60 Copyright (C) Microsoft Corp 1983-1987. All rights reserved. Run File [AMPE10.EXE]: List File [NUL.MAP]: Libraries [.LIB]: LINK : warning L4021: no stack segment C:\TASM>debug AMPE10.exe -g AX=1456 BX=0099 CX=0000 DX=0045 SP=0000 BP=0000 SI=0004 DI=0000 DS=14A4 ES=1494 SS=14A4 CS=14A5 IP=0027 NV UP EI PL ZR NA PE CY 14A5:0027 CC INT 3 -d 14A4:0000 14A4:0000 12 36 45 56 99 00 00 00-00 00 00 00 00 00 00 00 .6EV............ 14A4:0010 B8 A4 14 8E D8 B5 04 B1-04 8D 36 00 00 8A 04 8A ..........6..... 14A4:0020 5C 01 3A C3 72 08 8A 54-01 86 14 88 54 01 46 FE \.:.r..T....T.F. 14A4:0030 C9 75 EA FE CD 75 E0 CC-2B C0 8B F0 0B F6 74 0C .u...u..+.....t. 14A4:0040 8B DE D1 E3 03 1E 9A 16-8B 07 EB 02 2B C0 89 46 ............+..F 14A4:0050 F2 C4 5E F8 26 8B 47 08-89 46 F6 8B 5E 06 8B 07 ..^.&.G..F..^... 14A4:0060 8B 57 02 89 46 F8 89 56-FA C4 5E F8 26 83 7F 06 .W..F..V..^.&... 14A4:0070 00 74 0F 26 8B 5F 06 D1-E3 03 1E 96 16 8B 07 EB .t.&._..........
Understanding the Memory Dump
The memory dump after program execution shows the sorted array:
14A4:0000 12 36 45 56 99 00 00 00-00 00 00 00 00 00 00 00 .6EV............
The output 12, 36 45, 59, 99 confirms that the numbers have been successfully sorted in descending order.
By understanding and implementing this assembly program, we gain deeper insights into low-level data manipulation and the mechanics of sorting algorithms at the hardware interaction level.
Will you please explain the meaning of each instruction
PING ME ON WHATSAPP 7550171703
Hello tried running the above code. But could not the program
But could you english?
Yes I can English
.model small
.stack 100h
.data
array db 10 dup(?)
msg db “Enter SIX numbers”,0ah,0dh,’$’
msg0 db 0ah,0dh,”your acending numbers are”,0ah,0dh,’$’
max db 0
.code
mov ax,@data
mov ds,ax
mov ah,09h
mov dl,offset msg
int 21h
mov cx,6
l1:
mov si,offset array
mov ah,01h
int 21h
SUB AL,48
mov bl,al
l4:
cmp al,0
je l2
inc si
dec al
jmp l4
l2:
mov [si],bl
loop l1
mov ah,09h
mov dl,offset msg0
int 21h
mov si,offset array
mov cx,9
ll1:
mov al,[si]
cmp al,0
je ex1
mov ah,02h
mov dl,[si]
add dl,48
int 21h
ex1:
inc si
loop ll1
end
%include “io.inc”
section .data
data: dd 3,9,4,3,5,6,4,6,4,7
swap: db 0
rs: dd 0,0,0,0,0,0,0,0,0,0
section .text
global CMAIN
CMAIN:
start:
mov ebx,0
comparision:
mov eax,[data+ebx]
cmp eax,[data+ebx+4]
jb noswap
mov edx,[data+ebx+4]
mov [data+ebx+4],eax
mov [data+ebx],edx
mov byte[swap],1
noswap:
PRINT_DEC 4,eax
NEWLINE
add ebx,4
cmp ebx,36
jnz comparision
inc byte[swap]
cmp byte[swap],0
jnz start
mov [rs+ebx],eax
xor eax, eax
ret
i have problem in this code it is not showing requires result of bubble sorting of an array
Hey , i get an error at this , can u help me?
I have a problem here, can u help?
Don’t you think that program is wrong.
In the second iteration (up2) it does the same work. Then what’s the point of that. In first iteration also you won’t get smallest number. Please check the program once.
Will you pleas give a 8086 assembly language to find largest number in an array
mov al,0A
mov bl,0B
cmp al,bl
jg down
lea di,r
mov [di],al
hlt
down:
lea di,r
mov [di],al
hlt
r db ?
.model small
.stack 20
print macro msg
lea dx,msg
mov ah,09h
int 21h
endm
.data
dat1 db 01h,02h,03h,04h,05h,06h
count db 6
msg db 0dh,0ah,”The largest number is:$”
.code
start:mov ax,@data
mov ds,ax
mov ax,0000h
mov si,0000h
mov cl,count
x1:cmp al,dat1[si]
jg x2
x2:mov al,dat1[si]
inc si
loop x1
cmp al,0ah
jb num
add al,07h
num:add al,30h
print msg
mov dl,al
mov ah,02h
int 21h
mov ah,4ch
int 21h
end
start
hii can you help me
Program to Subtract two 8-bit numbers at
run-time i want codes
and there are many question it would be great i need it today plz
please explain the meaning of each instruction
i have installed Tasm but the cmd displays that debug is illegal command.please help
steps are
tasm filenames.asm
tlink filename.obj
and
td filename
NO its
tasm filename.asm
tlink filename
td filename
Thanks sir, it works..
Program to Subtract two 8-bit numbers at
run-time
can anyone help me with this with code in 8086
for user input