In the last tutorial, we have seen Spring Cloud: Exploring Spring Cloud Config Server (Native Method). In this tutorial, we will look into the GIT base method.
Spring cloud config allows you to have applications/micro-services configuration at a centralized place. Since we are working on spring micro-services. We may have hundreds of micro-services running together. Now we want to manage configuration for hundreds of those micro-services. It would be a big pain if we do it manually. Instead, we will use the config server provided by Spring Cloud to manage that configuration from a central place.
We are going to achieve the same goal here which we discussed in the earlier tutorial. But here we will move our configuration to a GIT repository.
TL;DR You can download whole project by clicking following link.
Creating a Git Repository with Common Configuration
For this tutorial, I have created a simple GIT repository on GitHub with the name ‘asm-cloud-config-git-tutorial’. In that repository, I will be adding the application.properties file which will contain our common configuration.
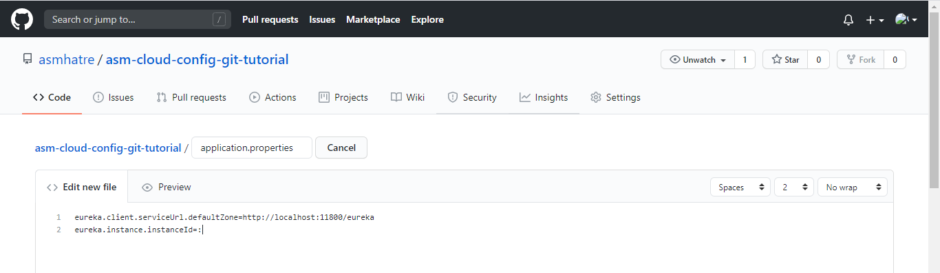
Here you can see that we have only one file in our GIT repository. That’s all that we need.
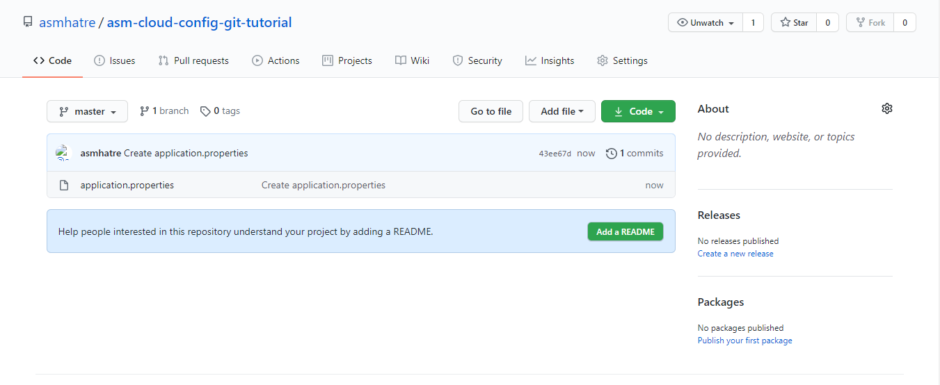
You may refer following link for this git repository.
https://github.com/asmhatre/asm-cloud-config-git-tutorial
Creating Config Server
Let’s set up our config server. For that, we will be creating a Maven Project. Our project structure is given below. We will be creating two files which are as follows:
- AsmCloudConfigGitApplication.java – Spring boot run–able file
- application.properties – Spring properties file
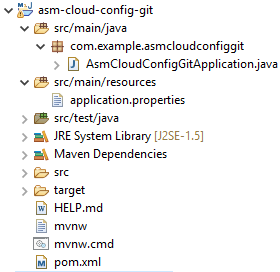
Adding Dependencies
Let’s add the required dependencies. Replace content in pom.xml file with the following content. Don’t forget to update the project using Maven > Update option. That will download all the necessary dependencies.
Note that we will require ‘spring-cloud-config-server‘ dependency for our server.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>asm-cloud-config-git</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>asm-cloud-config-git</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
<spring-cloud.version>Hoxton.SR6</spring-cloud.version>
<maven-jar-plugin.version>3.1.1</maven-jar-plugin.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Creating Spring Boot Application
Create file AsmCloudConfigGitApplication.java and add the following content. This file will serve as the entry point for our application. We will be adding @EnableConfigServer annotation on this class. This will enable the config server.
package com.example.asmcloudconfiggit;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.config.server.EnableConfigServer;
@SpringBootApplication
@EnableConfigServer
public class AsmCloudConfigGitApplication {
public static void main(String[] args) {
SpringApplication.run(AsmCloudConfigGitApplication.class, args);
}
}
Adding Properties
Under resources folder create a file with name application.properties and add the following content. Please note that here we have provided full link of our git repository which we have created in the earlier step.
server.port=8888
spring.cloud.config.server.git.uri=https://github.com/asmhatre/asm-cloud-config-git-tutorial
Updating Consumer Project
For consumer project we will be using application created in Spring Cloud: Creating REST Client Using Ribbon tutorial. As discussed earlier, we will be modifying that project to meet our requirements.
First, we will need to add ‘spring-cloud-starter-config‘ dependency in pom.xml. That dependency will add necessary components which will allow us to consume configurations from the config server.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
Now from application.properties file, we will remove Eureka Server URL and instance-id configuration. We have moved it to our common configuration.
spring.application.name=StudentConsumer1
server.port=11802
You can download the modified consumer project by clicking on the following link.
Updating Producer Project
For producer project, we will be using application created in Spring Cloud: Creating Student Service With Eureka. Now we will do similar changes in our producer application as well.
First we will add ‘spring-cloud-starter-config‘ dependency in pom.xml.
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
Then we will remove common configurations from application.properties.
spring.application.name=StudentProducer
server.port=0
You can download the modified producer project by clicking on the following link.
Eureka Server
Of-course we will need Eureka server as well. For that you can refer Setting Up Eureka Server Using Spring Cloud post you can download the following project and run it directly.
Testing it…
Now let’s start all of our applications. We will start with Eureka server first. Once Eureka server is up we will start Spring cloud config server. Then we will start producer and consumer application respectively. Once all applications are up, you can navigate to Eureka dashboard and check the status of running services.
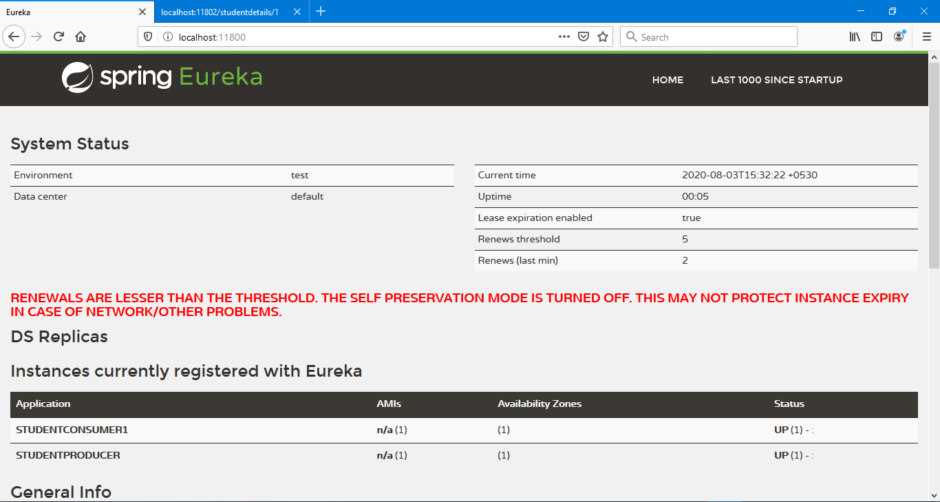
If you check logs of our producer or consumer application, you should see a line similar to which highlighted below. This line states that our application has contacted spring cloud config server for retrieving configuration.
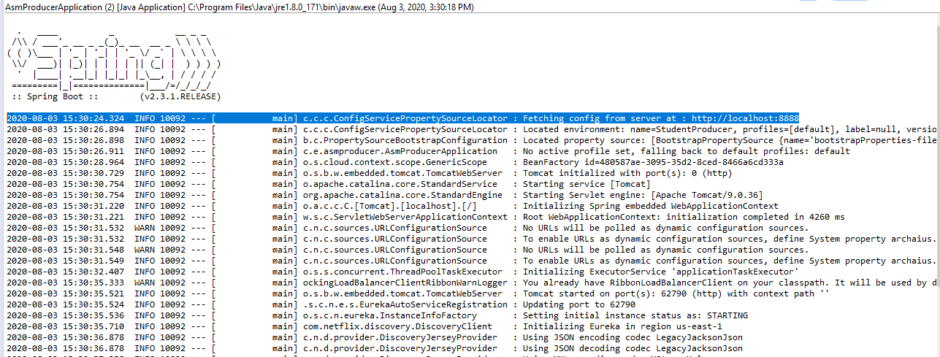
Let’s navigate to one of our endpoints and let’s check if we can able to access our service or not. If you navigate to ‘http://localhost:11802/studentdetails/1’ endpoint you should see output something similar to shown below. If you are getting this output that means that we have configured our spring application correctly.
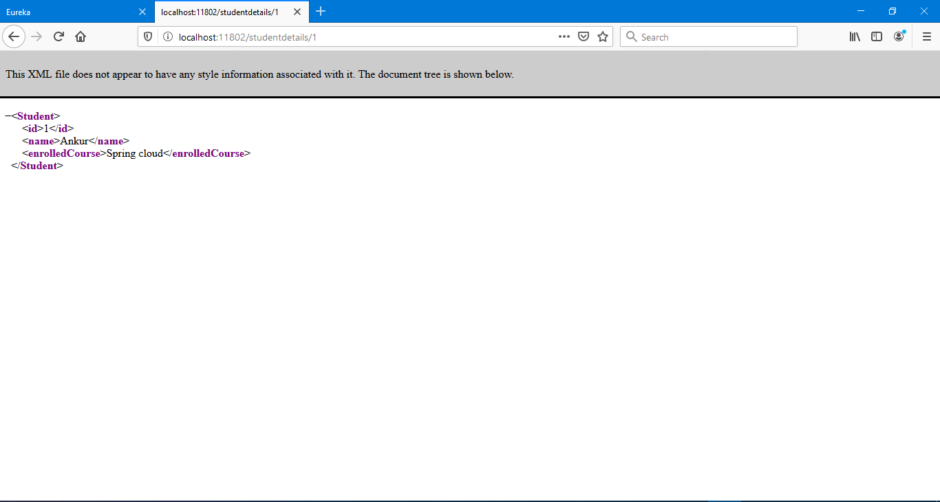
Downloads:
Git Repository: https://github.com/asmhatre/asm-cloud-config-git-tutorial
References:
- Spring Cloud Config Server Using Git Simple Example by JavaInUse
- Spring Cloud Configuration by Baeldung
- Spring Cloud Config Server with Git Integration by HowToDoInJava